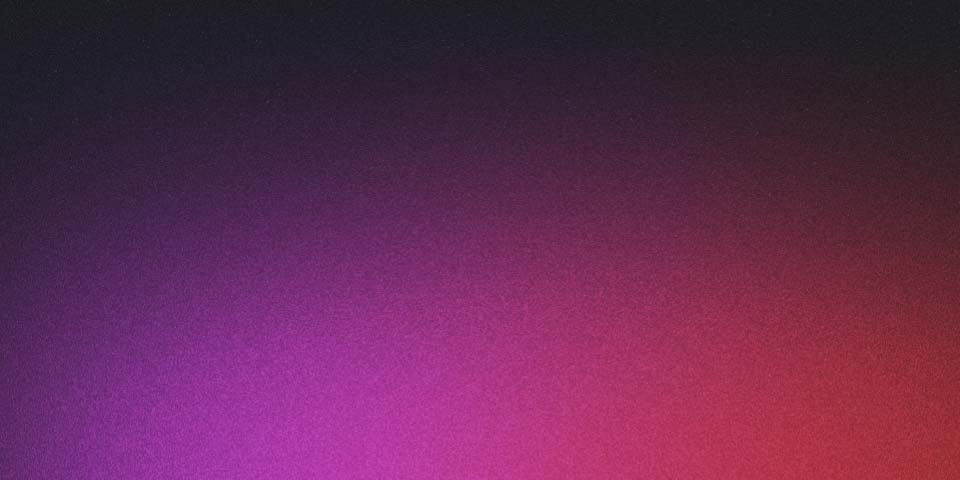
Fetch API
Using the Fetch API
The Fetch API provides an interface for fetching resources (including across the network). It is a more powerful and flexible replacement for XMLHttpRequest
Getting a resource
fetch("https://jsonplaceholder.typicode.com/posts/1")
.then((response) => response.json())
.then((json) => console.log(json));
Output
{
"id": 1,
"title": "...",
"body": "...",
"userId": 1
}
Listing all resources
fetch("https://jsonplaceholder.typicode.com/posts")
.then((response) => response.json())
.then((json) => console.log(json));
Output
[
{
"id": 1,
"title": "...",
"body": "...",
"userId": 1
},
/** ... */
{
"id": 100,
"title": "...",
"body": "...",
"userId": 1
}
]
Creating a resource
fetch("https://jsonplaceholder.typicode.com/posts", {
method: "POST",
body: JSON.stringify({
title: "foo",
body: "bar",
userId: 1,
}),
headers: {
"Content-type": "application/json; charset=UTF-8",
},
})
.then((response) => response.json())
.then((json) => console.log(json));
Output
{
"id": 101,
"title": "foo",
"body": "bar",
"userId": 1
}
Updating a resource
fetch("https://jsonplaceholder.typicode.com/posts/1", {
method: "PUT",
body: JSON.stringify({
title: "foo",
body: "bar",
userId: 1,
}),
headers: {
"Content-type": "application/json; charset=UTF-8",
},
})
.then((response) => response.json())
.then((json) => console.log(json));
Output
{
"id": 1,
"title": "foo",
"body": "bar",
"userId": 1
}
Patching a resource
fetch("https://jsonplaceholder.typicode.com/posts/1", {
method: "PATCH",
body: JSON.stringify({
title: "foo",
}),
headers: {
"Content-type": "application/json; charset=UTF-8",
},
})
.then((response) => response.json())
.then((json) => console.log(json));
Output
{
"id": 1,
"title": "foo",
"body": "bar",
"userId": 1
}
Deleting a resource
fetch("https://jsonplaceholder.typicode.com/posts/1", {
method: "DELETE",
});
Filtering resources
Basic filtering is supported through query parameters.
// This will return all the posts that belong to the first user
fetch("https://jsonplaceholder.typicode.com/posts?userId=1")
.then((response) => response.json())
.then((json) => console.log(json));